Connecting to the REST API:
Attention
This article does not describe all possible entity fields, a complete list is available in the documentation.
When using the API, your system must pass a simple basic authorization using administrator login and password, we recommend creating a special administrator in TMS for this purpose.
Below are examples of curl requests, the examples are for reference only, in real integrations you can use the handy http client of your programming language.
In real integrations, you need to consider possible loss of connectivity to the API, error handling, and other exceptional cases.
It is recommended to use the API to periodically synchronize your management system and TVIP TMS.
- HTTP methods:
GET - getting;
POST - create and modify;
DELETE - Delete.
When updating (changing) an entity, the json object can only contain the properties to be changed, fields that do not require changes can be omitted. When updating, it is necessary to indicate the id of the entity being changed in the json object; when creating the object, the id field is not used.
API authorization
When making calls to API methods it is necessary to use basic authorization, an example of its receipt and implementation is given below. It is important to set the Accept: application/json
field in header
.
# get Login - password for authorization
echo -ne login:password | base64
bG9naW46cGFzc3dvcmQK
# check by calling account list receiving method
c url -X GET --header 'Authorization: Basic bG9naW46cGFzc3dvcmQK' --header 'Accept: application/json' 'https://tms.example.com/api/provider/accounts'
Creating an account through the API
To create an account you need to generate json with account description, minimum data set required for the account:
- id:
user id in TVIP TMS, when creating a user, this parameter is omitted, when updating it is required.
- enable:
the property of the account that is responsible for the status of the service, if enable is set to false devices will be authorized, but services will not be available, this flag has to be used in your business logic to enable and disable the service.
- login:
username, unique within the provider, used to authorize devices by the user.
- pin_md5:
md5-encrypted user password is used to authorize devices by the user.
- fullname:
user name, will be displayed by the devices after user login.
- provider:
provider id.
The successful result of the command is a json object that contains all client properties including id of the user, this id will be used in the next steps.
curl \
--location \
--request POST 'http://tms.example.com/api/provider/accounts' \
--header 'Authorization: Basic bG9naW46cGFzc3dvcmQK' \
--header 'Content-Type: application/json' \
--data '{
"enabled": true,
"login": "user",
"pin_md5":"25d55ad283aa400af464c76d713c07ad",
"fullname":"First name Last name",
"provider" : 212
}'
# server response
{
"id": 4333,
"login": "user",
"remote_custom_field": null,
"fullname": "First name Last name",
"pin_md5": null,
"contract_info": null,
"main_address": null,
"account_desc": null,
"provider": 212,
"provider_dto": null,
"region_tag": null,
"region_dto": null,
"enabled": true,
"devices_per_account_limit": null
}
The result of the command can be seen in the administrator panel:
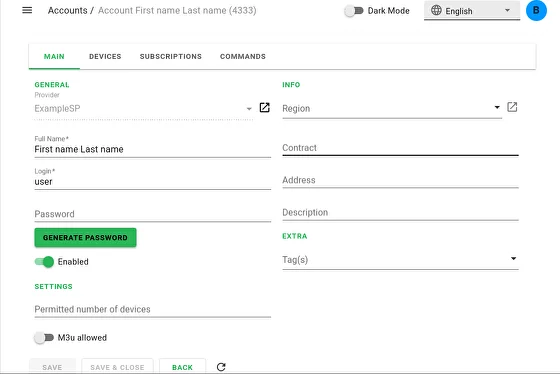
Adding a subscription to a user
Fields required to create a subscription:
- account:
user id in TMS (obtained in the previous step).
- start:
start date of the service.
- tarif:
tariff id for the service (you can create a tariff and specify its Id in the administrator panel).
Successful server response - json subscription object, the subscription id should be used to manage this subscription.
All client subscriptions can be obtained by :
/api/provider/account_subscriptions?account=4333
The response will contain a json response containing an array of subscriptions.
curl \
--location \
--request POST 'http://tms.example.com/api/provider/account_subscriptions' \
--header 'Authorization: Basic bG9naW46cGFzc3dvcmQK' \
--header 'Content-Type: application/json' \
--data '{
"account": 4333,
"start": "2020-05-19T00:00:00+0300",
"tarif": 69
}'
# server response
{
"start": "2020-05-19T00:00:00+0300",
"stop": null,
"tarif": 69,
"id": 13592,
"account": 4333,
"tarif_dto": null,
"account_dto": null
}
You can see the result of the command in the subscriptions section:
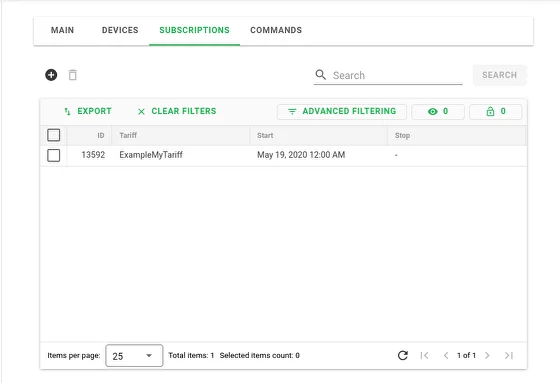
Subscription cancelling
To manage a subscription (for example, unsubscribe the user), you need to modify:
- id:
subscription id in TMS (obtained in the previous step).
- stop:
field for setting end date of the subscription.
A successful server response is a json subscription object with updated fields.
curl \
--location \
--request POST 'http:/tms.example.com/api/provider/account_subscriptions' \
--header 'Authorization: Basic bG9naW46cGFzc3dvcmQK' \
--header 'Content-Type: application/json' \
--data '{
"id" : 13592,
"stop": "2020-05-20T00:00:00+0300"
}'
# server response
{
"start": "2020-05-19T00:00:00+0300",
"stop": "2020-05-20T00:00:00+0300",
"tarif": 69,
"id": 13592,
"account": 4333,
"tarif_dto": null,
"account_dto": null
}
You can see the result of the command in your account settings:
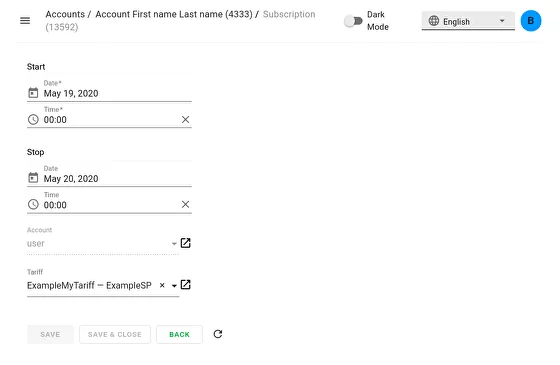
After there are no active subscriptions in account, device reports this message. The same message will appear when account is disabled.
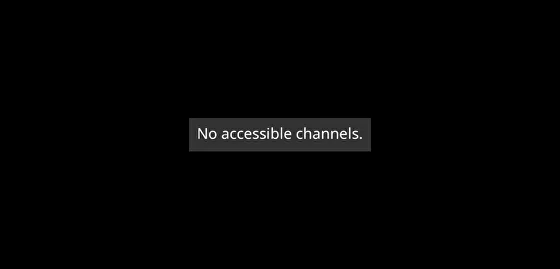
Account disconnection
Set the flag enabled: false
by id of the user in TMS
curl \
--location --request POST 'http://tms.example.com/api/provider/accounts' \
--header 'Authorization: Basic bG9naW46cGFzc3dvcmQK' \
--header 'Content-Type: application/json' \
--data '{
"enabled": false,
"id": 4333
}'
# ответ от сервера
{
"id": 4333,
"login": "user",
"remote_custom_field": null,
"fullname": "First name Last name",
"pin_md5": null,
"contract_info": null,
"main_address": null,
"account_desc": null,
"provider": 212,
"provider_dto": null,
"region_tag": null,
"region_dto": null,
"enabled": false,
"devices_per_account_limit": null
}
You can see the result of the command in your account settings:
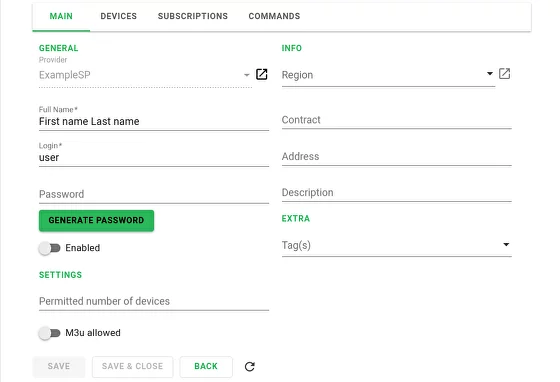