Billing API
Warning
Experimental functionality of the operator’s Personal account inside the TV application. The visual interface and API can be changed.
Changes in the document
04.03.2025
Updating the tariff
model:
added mandatory field
tms_id
added optional field
banner
, if not used the value should be set as nulladded optional field
background_color
, if not used the value should be set as nulladded optional field
benefits
, if not used value should be set as null or empty arrayremoved unused
prepaid
field from model description
Update the description of the SingleGroup
model:
added previously used but not documented fields archiveSubscriptions, futureSubscriptions
Update the description of the MultiGroup
model:
corrected error in model description (subscription field corrected to
subscriptions
)
Description
Serves for the implementation of the operator’s personal account inside the client applications, and it allows the following:
Read the current terms of service
Change the password to access the Account
Switch tariff plan
Subscribe to a tariff plan
Cancel the plan
Now Billing API support is only available in mobile applications.
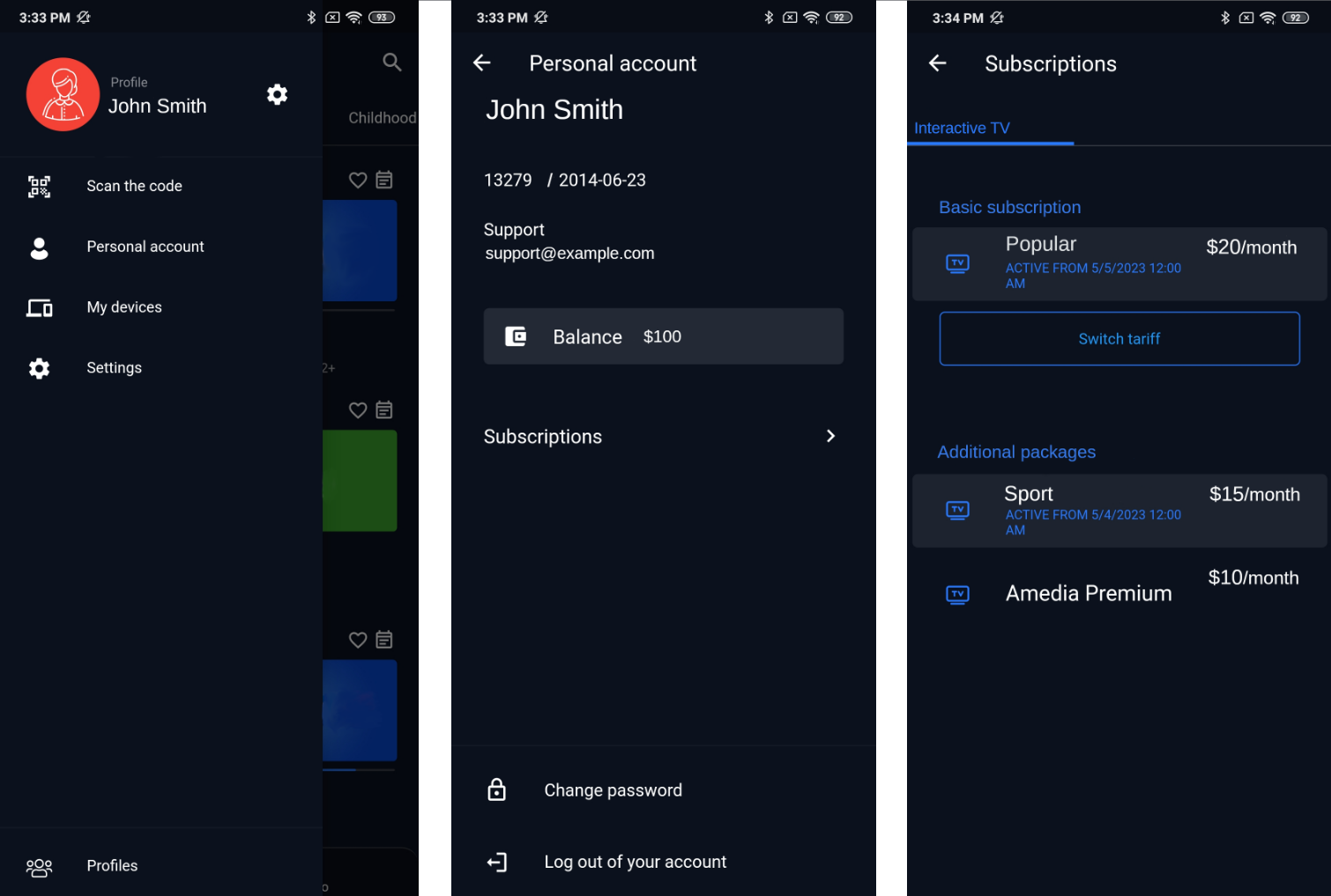
Changing the subscriptions may be limited. Below you may find the information how actions on tariff plans are limited.
The Billing API is a description of the interface between a user application and a billing operator.
Activation of Billing API
To enable the Billing API you need to:
Add
billing: true
to the /opt/tvip-tms/gateway/gateway-tvip-tms-tvip-tms-tvip-api/application-prod.yml file;
...
features:
billing: true
...
In the TMS Web interface, in the Provider settings, enable the Resolve Billing option, add a Url and token to access billing;
Restart the service
systemctl restart gateway-tvip-tms-tvip-api
.
Header variables available when requesting from TMS to operator billing
X-TMS-ACCOUNT-ID
- The ID of the account in the TMS whose request is being processed.
X-TMS-PROVIDER-ID
- The ID of the provider (an integer) whose request is being processed.
Accept-Language
- Locale of the client application.
X-AUTH-TOKEN
- Signature defined in the TMS provider settings. It is formed as follows:
private function createSign(Request $request):string{
$token = $request->getPathInfo();
$token = $token.$_ENV['TMS_TOKEN'];
if($request->headers->has('Content-Length') && $request->headers->get('Content-Length')>0){
$token = $token.$request->headers->get('Content-Length');
}
$md5 = md5($token);
return $md5;
}
General rules
Fields containing the date and time are transmitted in the format “Y-m-dTH:i:sO”, for example: 2023-05-03T10:55:11+0000
Responses to billing requests should contain correct HTTP codes, for example, 200 for successful requests, 500 for errors on the service side.
All requests are transmitted to TMS, and it will proxy them
as is
, just adding headersX-TMS-ACCOUNT-ID
,X-TMS-PROVIDER-ID
,X-AUTH-TOKEN
.All business logic and data processing in the billing and TMS are on the billing side, and the API only acts as an interface for service management from the client application.
Processed URLs to be implemented by the operator’s billing system
GET /api/cabinet - user account
POST /api/switch - change tariff plan
POST /api/subscribe - subscribe to a new subscription plan
POST /api/unsubscribe - unsubscribe
GET /api/tariff/{id} - detailed description of the tariff
POST /api/cabinet/password - Account password change
GET /api/cabinet
{
"contacts":"string"
"account":{
"id": 1,
"fullname":"John Doe",
"balance": "10 $",
"enabled": true,
"account_credentials": "132379-2014-01-01"
},
"services":[
{
"type": "iptv",
"title": "Interactive TV",
"description" "TV with timeshift and catch-up"
"groups":[
{
"title": "Basic subscription",
"type": "SINGLE",
"offers":[
{
"from": "2023-06-01T00:00:00+0300",
"tariff":{
"id": 2,
"tms_id": 22,
"banner": null,
"name": "Standard channels",
"cost": "2 $",
"period": "monthly",
"description": "",
"channel_count": 150,
"background_color": "#000000",
"benefits": ["TOP 250","Cooking with masters"]
}
}
],
"subscription":{
"id": 19589,
"from": "2022-11-03T00:00:00+0300",
"to": null,
"cancel_date": null,
"tariff":{
"id": 1,
"tms_id": 11,
"banner": "http://example.com/banner.png",
"name": "Basic channels",
"cost": "1 $",
"period": "monthly",
"description": "",
"channel_count": 100,
"background_color": null,
"benefits": null
}
},
"archiveSubscriptions":[],
"futureSubscriptions":[],
},
{
"title": "Premium packages",
"type": "MULTI",
"offers":[],
"subscriptions":[]
}
]
}
]
}
The object cabinet
contains the following fields:
- cabinet.contacts:
The line, for example, there could be a support email address or phone number.
- cabinet.account:
The account object contains information about the subscriber: name of the contract holder, contract details, current balance, the flag
enabled
indicates whether the subscriber’s services are enabled.- cabinet.services:
The array of services that can be managed through Personal Area in the application is currently supported only by
type
iptv.- service.groups:
The service contains groups (
groups
), which in turn can havetype
:SINGLE - for plans that can replace each other and cannot be active simultaneously, e.g., ‘Basic Tariff’ and ‘Advanced Tariff’.
MULTI - for plans that may be active simultaneously, usually they include movie or sports channels.
- group.offers:
Common for both types of groups, the field contains offers for subscribing or switching (for SINGLE type) to tariff plans:
from
- the date from which it is proposed to subscribe (change subscription)tariff
- the tariff to which the subscription is offered
- group.subscription:
Specific field for SINGLE group type, can be
null
or shows the current subscription:id
- subscription identifier, it is used as an argument in unsubscribe and unsubscribe methodsfrom
- start date of the subscriptionto - end date of subscription, null - no end date defined
cancel_date
- the date from which it is possible to end the subscription (unsubscribe), if set to null - it’s impossible to cancel a subscription through the LC.tariff
- description of the subscription plan
- group.subscriptions:
Specific field for MULTI group type, array subscriptions, has the same structure as for SINGLE group
- group.archiveSubscriptions:
Array of past subscriptions, specific to SINGLE groups
- group.futureSubscriptions:
Array of scheduled subscription changes, allows you to cancel a scheduled subscription
- tariff:
Tariff object, describes the properties of the tariff plan:
id
- tariff identifier in billing, used in subscription methodstms_id
- integer field, tariff identifier in TMSbanner
- banner for the tariff, optional field, link to an image with dimensions 1020px x 640pxname
- the name of the tariff plan.cost
- the cost of the tariff plan, it is a simple string, it is allowed to use the accepted notation for the region of service provision, for example, “20 EUR” or “$ 20”.period
- the billing period of the service, use the wording understandable to the subscriber, for example “per day”, “per year”.description
- a description of the tariff plan, describe the features or describe the benefits.channel_count
- an integer field, specify the number of channels in the tariff plan.background_color
- color of the card, if not specified, the application will generate the color automatically.benefits
- array of strings advertising the tariff plan, total number of characters not more than 70, number of characters of an individual line not more than 35 characters. Null or empty array is treated as an unset parameter
Note
Recommendations for design
Color background_color
:
Any color is acceptable, but keep in mind that the text is always white.
Avoid colors that are too bright or too light.
Text benefits
:
The maximum length of one benefit is up to 35 characters.
The total number of characters in all benefits is a maximum of 70 characters.
Tariff Description:
name
(tariff title): up to 45 characters.description
: up to 300 characters.
The banner
design
Banner size: 1020×640 px.
The banner placed in the tariff card is duplicated on the tariff description page.
POST /api/switch
Server request model
{
"subscription_id": 19589,
"tariff_id": 2,
"from": "2023-06-01T00:00:00+0300"
}
Change subscription method, used only for SINGLE groups, the application generates a request based on the selected offer
:
subscription_id
- identifier of the subscription to be changed
tariff_id
- identifier of the tariff in the selected subscriberoffer
from
- the date of change of the tariff plan in the subscription, specified in the selected by the subscriberoffer
Server response model
{
"status": 200,
"message": null,
"subscription": {
"id": 19589,
"from": "2023-06-01T00:00:00+0300",
"to": null,
"cancel_date": null,
"tariff":{
"id": 2,
"tms_id": 22,
"banner": null,
"name": "Standard channels",
"cost": "2 $",
"period": "monthly",
"description": "",
"channel_count": 150,
"background_color": null,
"benefits": null
}
}
}
Response fields
status
- response code 200 should be set in case of correct execution of the request, in other cases you should specify 500.
message
- message to the subscriber, not used at the moment.
subscription
- model of the generated subscription (group.subscription
)
Note
At the moment only HTTP code is processed. HTTP response code should be 200 if the request is executed without errors. The HTTP response code should be 500 if the request is not executed.
POST /api/subscribe
Server request model
{
"tariff_id": 2,
"from": "2023-06-01T00:00:00+0300"
}
Making a new subscription in the group:
tariff_id
- identifier of the tariff in the selected subscriberoffer
from
- the date of change of the tariff plan in the subscription, specified in the selected by the subscriberoffer
Server response model
{
"status": 200,
"message": null,
"subscription": {
"id": 19589,
"from": "2023-06-01T00:00:00+0300",
"to": null,
"cancel_date": null,
"tariff":{
"id" 2,
"tms_id:" 22,
"banner": null,
"name": "Standard channels",
"cost": "2 $",
"period": "monthly",
"description": "",
"channel_count": 150,
"background_color": null,
"benefits": null
}
}
}
Response fields
status
- response code 200 should be set in case of correct execution of the request, in other cases you should specify 500.
message
- message to the subscriber, not used at the moment.
subscription
- model of the generated subscription (group.subscription
)
Note
At the moment only HTTP code is processed. HTTP response code should be 200 if the request is executed without errors. The HTTP response code should be 500 if the request is not executed.
POST /api/unsubscribe
Server request model
{
"subscription_id": 100,
"to": "2023-07-01T00:00:00+0300"
}
Unsubscribe is possible only if the field subscription.cancel_date
is defined, in which case the client will have a button that will generate a request:
subscription_id
- Subscription IDto - end date of subscription
Server response model
{
"status": 200,
"message": null,
"subscription": {
"id": 100,
"from": "2023-06-01T00:00:00+0300",
"to": "2023-07-01T00:00:00+0300",
"cancel_date": null,
"tariff":{
"id": 2,
"tms_id": 22,
"banner": null,
"name": "Standard channels",
"cost": "2 $",
"period": "monthly",
"description": "",
"channel_count": 150,
"background_color": null,
"benefits": null
}
}
}
Response fields:
status
- response code 200 should be set in case of correct execution of the request, in other cases you should specify 500.
message
- message to the subscriber, not used at the moment.
subscription
- the modified subscription model (group.subscription
)
Note
At the moment only HTTP code is processed. HTTP response code should be 200 if the request is executed without errors. The HTTP response code should be 500 if the request is not executed.
GET /api/tariff/{id}
Request for details of the tariff plan with the identifier {id}
Server response model
{
"tariff": {
"id": 10,
"tms_id": 22,
"banner": null,
"name": "Amedia Premium",
"cost": "99 $",
"period": "monthly",
"description": "",
"channel_count": 1,
"background_color": null,
"benefits": null
},
"channels": [
{
"name": "Amedia Premium HD",
"logo_url": "https://example.com/logo.png",
"description": "",
"number": 322,
"categories": [
2,
3
]
}
],
"categories": {
"1": "News",
"2": "Movies",
"3": "HD Channels",
}
}
Response fields:
tariff
- the tariff model described above.channels
- an array of channels included in the tariff plan.categories
- a directory of categories.
POST /api/cabinet/password
Method of changing the password from the Subscriber’s account in TMS
Server request model
{
"old_password": "0512f08120c4fef707bd5e2259c537d0"
"password": "5f4dcc3b5aa765d61d8327deb882cf99"
}
Field Description:
old_password
- the current password entered by the user.
password
- the new password entered by the user.
Your backend, based on the data transmitted, should change the password or refuse to change the password
Server response model
{
"status": 200,
"message": null,
}
Field Description:
status
- 200 in case of password change, 500 in other cases.
message
- message to the subscriber, not used at the moment.
Note
At the moment only HTTP code is processed. HTTP response code should be 200 if the request is executed without errors. The HTTP response code should be 500 if the request is not executed.
Description of models
Cabinet
Property |
type |
nullable |
---|---|---|
contacts |
string |
no |
account |
Account model |
no |
services |
Service[] |
no |
Account model
Property |
type |
nullable |
---|---|---|
id |
int |
no |
fullname |
String |
no |
balance |
String |
yes |
enabled |
bool |
no |
account_credentials |
String |
yes |
Service model
Property |
type |
nullable |
---|---|---|
type |
String |
no |
title |
String |
no |
description |
String |
yes |
groups |
GroupInterface[] |
no |
GroupInterface
Property |
type |
nullable |
---|---|---|
title |
String |
no |
type |
const: SINGLE |
no |
offers |
Offer[] |
no |
subscription |
Subscription |
yes |
archiveSubscriptions |
Subscription[] |
no, empty or filled array required |
futureSubscriptions |
Subscription[] |
no, empty or filled array required |
Property |
type |
nullable |
---|---|---|
title |
String |
no |
type |
const: MULTI |
no |
offers |
Offer[] |
no |
subscriptions |
Subscription[] |
no, empty or filled array required |
TariffInterface
Property |
type |
nullable |
---|---|---|
id |
int |
no |
tms_id |
int |
no |
banner |
string |
yes |
background_color |
string |
yes |
benefits |
string[] |
yes |
name |
String |
no |
cost |
String |
no |
description |
String |
yes |
channel_count |
int |
no |
Offer
Property |
type |
nullable |
---|---|---|
tariff |
TariffInterface |
no |
from |
String |
no |
Subscription
Property |
type |
nullable |
---|---|---|
id |
int |
no |
from |
String |
no |
to |
String |
yes |
cancel_date |
String |
yes |
tarif |
TariffInterface |
no |